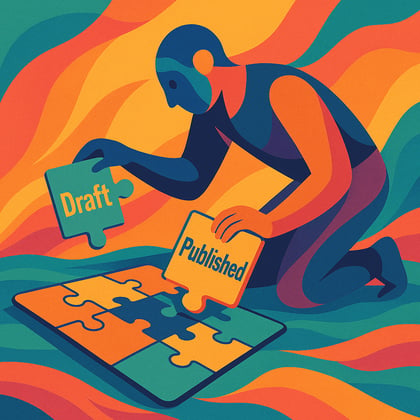
How Storyblok Handles Content Versioning
A practical guide to how Storyblok handles draft and published content, with examples of API usage a
Optimizely Most Valued Professional (OMVP), Subject Matter Expert (SME), CMS and Commerce certified
Contact MeHere I have an URL with a query string:
https://hiddenfoundry.com/page?id=123
I don't like this query string, I want a friendly segment instead:
https://hiddenfoundry.com/page/123
How do I go about making this change in Optimizely?
Optimizely provides an interface called IPartialRouter which allows you to do just this. Here's what the complete code looks like:
public class YourPagePartialRouter : IPartialRouter<YourPage, YourPage>
{
public PartialRouteData GetPartialVirtualPath(YourPage content,
string language,
RouteValueDictionary routeValues,
RequestContext requestContext)
{
return null;
}
public object RoutePartial(YourPage content, SegmentContext segmentContext)
{
var segmentPair = segmentContext.GetNextValue(segmentContext.RemainingPath);
segmentContext.RouteData.Values["id"] = segmentPair.Next;
segmentContext.RemainingPath = segmentPair.Remaining;
return content;
}
}
Let's break this down.
A. public class YourPagePartialRouter : IPartialRouter<YourPage, YourPage>
Implement a class inheriting from IPartialRouter. You'll notice we need to specify two generic parameters: IPartialRouter<TContent, TRoutedData>
In our example, both TContent and TRoutedData are the same.
By inheriting the interface you must implement two methods. GetPartialVirtualPath and RoutePartial.
B. GetPartialVirtualPath()
- We don't need this.
GetPartialVirtualPath() from the full example you can see we return a null. We don't care about generating a url when requested since we are treating the last segment as a query parameter.
C. RoutePartial()
- Breaking down the magic
Grab the next segment pair which contains the next segment and also the remaining path.
var segmentPair = segmentContext.GetNextValue(segmentContext.RemainingPath);
Assign the next segment to our routed parameter. In our example, segmentPair.Next would equal to "123"
segmentContext.RouteData.Values["id"] = segmentPair.Next;
Assign what's left the the remaining path for the next pass when it iterates over.
Note we don't not pass an empty string here. This would effectively be instructing the partial router to ignore the remaining path, meaning any combination of paths after what we've already processed would resolve to this page. Not good for seo!
segmentContext.RemainingPath = segmentPair.Remaining;
return content;
A practical guide to how Storyblok handles draft and published content, with examples of API usage a
Have you taken the time to investigate what SaaS actually offers before introducing it to your busin
Overcomplicated requirements are slowing you down. Here’s how trimming the excess can help you launc
I discuss our experiences not validating business features early which result in delayed projects
Best practice of managing your Optimizely nuget packages
Demystify Image Resizing on the edge for your Optimizely solution
Critical parts of an upgrade can help you understand the different stages of an upgrade. Let's discu
Optimizely Customers now have a choice between using CMS on either PaaS or SaaS
SaaS Core is Optimizely's latest CMS offering as part of its composable architecture
We describe Optimizely's newest Content as a Service offering Optimizely Graph and how you should be
Straightforward RSS feed generation for your Optimizely solution with this NuGet package
Headless vs Traditional doesn't have to be a hard choice. An Optionality approach gives you the best
We are proud to announce Optimizely has awarded Hidden Foundry with Specializations
We discuss our experiences of working with Navico to refactor their single site into a multi-site so
We summerize our experience of working with FirstMile and helping them move over to Optimizely Comme
Grasp ODP and how it's AI and data consolidation enables insight driven conversions
We outline the benefits of upgrading your Optimizely solution to ASP.NET Core
Expand content area properties to load nested contact areas recursively to a customizable level
Redirect the user elsewhere instead of the default Optimizely login page when attempting to access a
Learn to use CLI and dotnet command to create new cms / commerce projects and perform admin actions.